Unlocking the Power of Mako Templates: Integrating with Django for Seamless Web Development
As web developers, we're well aware of the necessity of a web framework that efficiently generates HTML pages dynamically using server-side scripting. Django, in its approach, initially introduced Django Template Language (DTL), which operates with a new templating syntax. Additionally, Django seamlessly integrates with Jinja2, a faster and memory-efficient alternative to DTL.
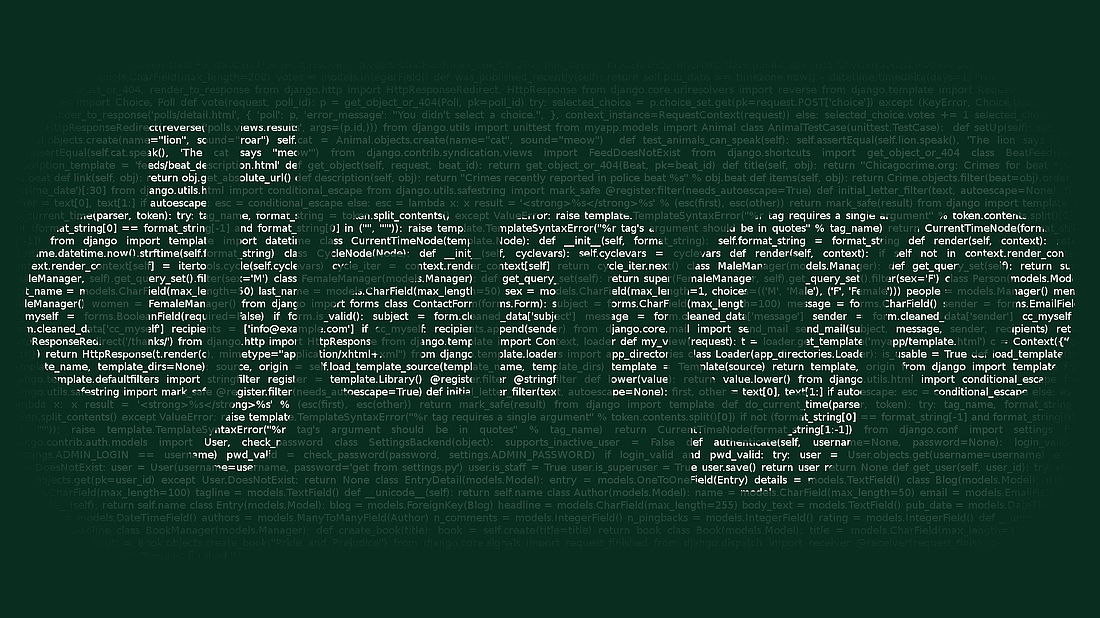
Recently, while incorporating Mako templates into a Django project, I noticed a dearth of resources on integrating Mako with Django. Hence, I decided to share my experience and the minimal solution I devised with fellow developers interested in supporting Mako templates in their projects.
In this post, I'll walk you through the detailed process of integrating a custom template library—in this case, Mako—with your Django project. If you prefer to dive straight into the solution, feel free to skip to the Gist files I've provided, which are extensively documented to aid your understanding.
However, if you're still here, get ready for a deep dive into the nitty-gritty of integrating a new template library. Note that while I'll strive to explain every step comprehensively, some concepts or configurations may not be directly related to the setup process. In such cases, don't hesitate to refer to Django's documentation for detailed explanations.
Let's kick off by installing the necessary requirements for your chosen template library. For Mako, simply include the following line in your requirements file:
mako==1.0.6
Pinning Mako's version isn't necessary.
Configuration
While it might not be the most exciting part, configuring your templates backend is crucial. Django provides the TEMPLATES
setting for this purpose, which consists of a list of configuration dictionaries, each representing an engine. The default value for TEMPLATES
is an empty list, implying no templates configured.
Each configuration specifies the backend engine, its options, and the directories containing templates. Below is a snippet illustrating various configuration possibilities:
# ...
TEMPLATES = [
{
'BACKEND': 'backends.mako.MakoTemplates',
'NAME': 'mako',
'DIRS': [
os.path.join(BASE_DIR, 'templates/mako'),
],
},
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [
'/home/html/templates/lawrence.com',
'/home/html/templates/default',
],
},
{
'BACKEND': 'django.template.backends.jinja2.Jinja2',
'DIRS': [os.path.join(BASE_DIR, 'templates/jinja2')],
'OPTIONS': {
# Any engine-specific options.
},
},
]
# ...
- The first item configures our custom backend (in this case, Mako) by specifying the backend engine's path and providing a unique name. This configuration includes the directories where Mako templates reside.
- The second item configures the DTL backend, indicating specific directories for template lookup.
- The third item configures the Jinja2 backend, demonstrating the use of additional options.
Engine Selection
Understanding how Django selects template engines is crucial. Django employs various methods to determine the appropriate engine for rendering a specific template:
- Explicit Selection: You can explicitly specify the engine for rendering a template in function-based or class-based views, though this approach comes with its drawbacks.
- Backend-Specific Paths: This method involves organizing templates into specific folders linked with corresponding backend configurations.
- Trial and Error: Django iterates over the configured engines, attempting to locate the template until successful. Hence, the order of engines in the
TEMPLATES
list matters.
The Engine
The engine acts as an intermediary between Django and the template library, responsible for retrieving and compiling templates. Here's a simplified version of a Mako engine:
class MakoEngine(object):
def __init__(self, **options):
# Initialization logic
def get_template(self, name):
# Retrieve template logic
def from_string(self, template_code):
# Compile template logic
The Template Object
Template objects returned by backends must contain a render
method responsible for rendering the template given a context and request. Here's a basic implementation:
class Template(object):
def __init__(self, template):
# Initialization logic
def render(self, context=None, request=None):
# Render template logic
The Django Backend API
To integrate a new template system with Django, you need to implement a custom template backend, inheriting from django.template.backends.base.BaseEngine
. Here's a simplified example for Mako:
class MakoTemplates(BaseEngine):
app_dirname = 'mako'
def __init__(self, params):
# Initialization logic
def from_string(self, template_code):
# Compile template logic
def get_template(self, template_name):
# Retrieve template logic
Testing
Finally, testing your integration is crucial. Run the Django management console and execute simple functionality tests. Here's a basic example:
python manage.py shell
from django.template import engines
mako_engine = engines['mako']
template = mako_engine.from_string('Hello ${name}!')
context = {'name': 'Ahmed'}
template.render(context)
With these steps, you've successfully integrated a custom template library into your Django project. For a comprehensive solution, refer to the provided Gist. Simply integrate the code into your project, and you're good to go!
Love,
Ahmed Jazzar